ADDING THE CODE TO THE FORMS
Now that we have all the forms prepared, we need to add the underlying code. We will
first start with the About form and the Definition forms. To add code double click on the
object you would like to add code to. In this case we need to add code to the Exit
Buttons. So, go to the About form and double click on the Exit button. When you do this
a programming window should appear with a function all set up and ready to insert code
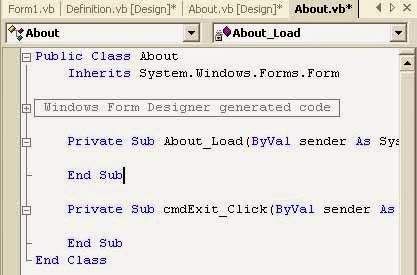
THE SOURCE CODE:
ABOUT FORM:
EXIT BUTTON:
Private Sub cmdExit_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdExit.Click
Close() ' unload the current form
End Sub
DEFINITION FORM:
EXIT BUTTON:
Private Sub cmdExit_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdExit.Click)
Close() ' unload the current form
End Sub
STACK FORM:
EXIT BUTTON:
Private Sub cmdExit_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdExit.Click
End
End Sub
Now we need to add the code for the objects of the Stack form.
STACK FORM CODE:
OPTION EXPLICIT:
Public Index As Integer
Const STACK_LENGTH = 9
‘Note: This is where all the global variables go. The global variables can be accessed
‘anywhere in the program. Option Explicit also provides strong type checking in VB,
‘and makes the programmer declare any variables before they are used
Private Sub frmStack_Load(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles MyBase.Load
Index = 0
End Sub
Command Buttons:
PUSH:
Private Sub cmdPush_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdPush.Click
Push()
End Sub
POP:
Private Sub cmdPop_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdPop.Click
Pop()
End Sub
PEEK:
Private Sub cmdPeek_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdPeek.Click
Peek()
End Sub
INITALIZE
Private Sub cmdInitalize_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdInitalize.Click
Initalize()
End Sub
Text Box Code:
Private Sub txtEnterString_KeyPress(KeyAscii As Integer)
Const ENTERKEY = 13
If KeyAscii = ENTERKEY Then
Push()
End If
End Sub
Push
Public Function Push()
If txtEnterString.Text = "" Then
Beep()
MsgBox("Nothing Entered to Push")
ElseIf Index <= STACK_SIZE Then
lstStack.Items.Insert(0, txtEnterString.Text)
txtEnterString.Text = ""
Index = Index + 1
Else
Beep()
MsgBox("Stack is Full")
End If
End Function
Pop
Public Function Pop()
If Index = 0 Then
Beep()
MsgBox("Stack is Empty")
Else
txtPoppedString.Text = lstStack.Items.Item(0)
lstStack.Items.RemoveAt(0)
Index = Index - 1
End If
End Function
Peek
Public Function Peek()
If Index = 0 Then
MsgBox("Stack is empty")
Else
txtTopString = lstStack.Items.Item(0)
End If
End Function
Initialize
Public Function Initialize()
If Index = 0 Then
Beep()
MsgBox("Stack is Clear")
Else
While Index > 0
lstStack.Items.RemoveAt(0)
Index = Index - 1
End While
End If
txtPoppedString.Text = ""
txtTopString.Text = ""
End Function
Menu_Click
Private Sub mnuDefinition_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs)Handles mnuDefinition.Click
Dim x As frmDefinition = New frmDefinition()
x.Show()
End Sub
Private Sub mnuAbout_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles mnuAbout.Click
Dim y As frmAbout = New frmAbout()
y.Show()
End Sub
Now you should be able to execute the program. If there are errors in execution then you
may have some typing mistakes. Double check your code and try again. If you have any
questions go to the VB help and search for the control that you are having problems with.
You will get better results if you search for the control using the same spelling that Microsoft did, i.e. mainmenu instead of main menu.
Now that we have all the forms prepared, we need to add the underlying code. We will
first start with the About form and the Definition forms. To add code double click on the
object you would like to add code to. In this case we need to add code to the Exit
Buttons. So, go to the About form and double click on the Exit button. When you do this
a programming window should appear with a function all set up and ready to insert code
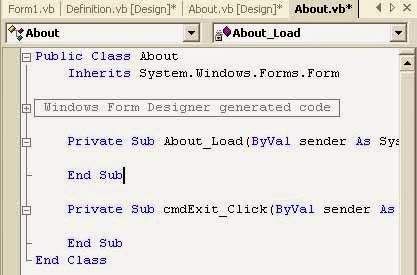
ABOUT FORM:
EXIT BUTTON:
Private Sub cmdExit_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdExit.Click
Close() ' unload the current form
End Sub
DEFINITION FORM:
EXIT BUTTON:
Private Sub cmdExit_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdExit.Click)
Close() ' unload the current form
End Sub
STACK FORM:
EXIT BUTTON:
Private Sub cmdExit_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdExit.Click
End
End Sub
Now we need to add the code for the objects of the Stack form.
STACK FORM CODE:
OPTION EXPLICIT:
Public Index As Integer
Const STACK_LENGTH = 9
‘Note: This is where all the global variables go. The global variables can be accessed
‘anywhere in the program. Option Explicit also provides strong type checking in VB,
‘and makes the programmer declare any variables before they are used
Private Sub frmStack_Load(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles MyBase.Load
Index = 0
End Sub
Command Buttons:
PUSH:
Private Sub cmdPush_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdPush.Click
Push()
End Sub
POP:
Private Sub cmdPop_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdPop.Click
Pop()
End Sub
PEEK:
Private Sub cmdPeek_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdPeek.Click
Peek()
End Sub
INITALIZE
Private Sub cmdInitalize_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles cmdInitalize.Click
Initalize()
End Sub
Text Box Code:
Private Sub txtEnterString_KeyPress(KeyAscii As Integer)
Const ENTERKEY = 13
If KeyAscii = ENTERKEY Then
Push()
End If
End Sub
Push
Public Function Push()
If txtEnterString.Text = "" Then
Beep()
MsgBox("Nothing Entered to Push")
ElseIf Index <= STACK_SIZE Then
lstStack.Items.Insert(0, txtEnterString.Text)
txtEnterString.Text = ""
Index = Index + 1
Else
Beep()
MsgBox("Stack is Full")
End If
End Function
Pop
Public Function Pop()
If Index = 0 Then
Beep()
MsgBox("Stack is Empty")
Else
txtPoppedString.Text = lstStack.Items.Item(0)
lstStack.Items.RemoveAt(0)
Index = Index - 1
End If
End Function
Peek
Public Function Peek()
If Index = 0 Then
MsgBox("Stack is empty")
Else
txtTopString = lstStack.Items.Item(0)
End If
End Function
Initialize
Public Function Initialize()
If Index = 0 Then
Beep()
MsgBox("Stack is Clear")
Else
While Index > 0
lstStack.Items.RemoveAt(0)
Index = Index - 1
End While
End If
txtPoppedString.Text = ""
txtTopString.Text = ""
End Function
Menu_Click
Private Sub mnuDefinition_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs)Handles mnuDefinition.Click
Dim x As frmDefinition = New frmDefinition()
x.Show()
End Sub
Private Sub mnuAbout_Click(ByVal sender As System.Object, ByVal e As
System.EventArgs) Handles mnuAbout.Click
Dim y As frmAbout = New frmAbout()
y.Show()
End Sub
Now you should be able to execute the program. If there are errors in execution then you
may have some typing mistakes. Double check your code and try again. If you have any
questions go to the VB help and search for the control that you are having problems with.
You will get better results if you search for the control using the same spelling that Microsoft did, i.e. mainmenu instead of main menu.
0 comments:
Post a Comment